[Flask] API 만들기
GET, POST 요청타입 - Remind
* GET
→ 통상적으로! 데이터 조회(Read)를 요청할 때 예) 영화 목록 조회
→ 데이터 전달 : URL 뒤에 물음표를 붙여 key=value로 전달
→ 예: google.com?q=북극곰
* POST
→ 통상적으로! 데이터 생성(Create), 변경(Update), 삭제(Delete) 요청 할 때 예) 회원가입, 회원탈퇴, 비밀번호 수정
→ 데이터 전달 : 바로 보이지 않는 HTML body에 key:value 형태로 전달
GET 요청 API 만들기
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h1>나의 첫 웹페이지!</h1>
<button>버튼을 만들자</button>
</body>
</html>
우선 이 index.html HTML 파일에 JQuery 부터 추가해보자
▼
참조
2022.12.26 - [𝐃𝐞𝐯𝐞𝐥𝐨𝐩/JQuery] - [JQuery] 복습하며 정리하기 (1)
[JQuery] 복습하며 정리하기 (1)
JQuery란? 우선 JQuery가 뭔지 알려주겠다. JQuery는 Javascript를 사용하기 쉽게 미리 작성해둔 것 즉 '라이브러리'로 칭한다. CSS 에서는 class= " " 로 지칭을 한다면, Javascript 에서는 id= " " 로 지칭을 한다.
jrogrammer.tistory.com
JQuery 임포트 (코드스니펫)
▼
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
해당 구문을 head 안에 넣어준다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
</head>
<body>
<h1>나의 첫 웹페이지!</h1>
<button>버튼을 만들자</button>
</body>
</html>
기입해줬다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script>
function hey() {
}
</script>
</head>
<body>
<h1>나의 첫 웹페이지!</h1>
<button>버튼을 만들자</button>
</body>
</html>
그 다음 위 코드 처럼
<script>
function hey() {
}
</script>
head 에 script 를 하나 넣고 function hey() {} 를 넣어주자
그 다음
<button onclick="hey()">버튼을 만들자</button>
버튼 코드 안에 onclick="hey()"를 넣어주자
이 코드들은 버튼을 누르면 function 에 있는 기능들이 동작하도록 만들어 준 것이다.
그렇다면 script 안에 있는 function 안에는 무엇을 넣었었느냐?
GET 요청 확인 AJAX 코드를 넣었었다.
참조
2022.12.27 - [𝐃𝐞𝐯𝐞𝐥𝐨𝐩/Ajax] - [Ajax] (1) 시작하기
GET 요청 확인 AJAX 코드 (코드스니펫)
▼
$.ajax({
type: "GET",
url: "여기에URL을입력",
data: {},
success: function (response) {
console.log(response)
}
})
url 넣어서
<script>
function hey() {
$.ajax({
type: "GET",
url: "/test?title_give=봄날은간다",
data: {},
success: function (response) {
console.log(response)
}
})
}
</script>
이렇게 변경해준다.
여기서
url: "/test?title_give=봄날은간다",
을 한번 헤집어 보자
/test? => test 라는 창구에서
title_give => title_give 라는 이름으로
봄날은간다 => 봄날은간다라는 데이터를 내가 가져갈게
라는 url 형식이다.
success: function (response) {
console.log(response)
그리고 성공한다면 너가 주는 데이터를 콘솔에다가 찍어볼게 라는 코드이다.
그렇다면!?
/test? 라는 창구는 어떻게 만드냐?
app.py 로 돌아와서
GET 요청 API코드(코드스니펫)
▼
@app.route('/test', methods=['GET'])
def test_get():
title_receive = request.args.get('title_give')
print(title_receive)
return jsonify({'result':'success', 'msg': '이 요청은 GET!'})
GET 요청 API 코드를
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def home():
return render_template('index.html')
@app.route('/test', methods=['GET'])
def test_get():
title_receive = request.args.get('title_give')
print(title_receive)
return jsonify({'result':'success', 'msg': '이 요청은 GET!'})
if __name__ == '__main__':
app.run('0.0.0.0', port=5000, debug=True)
해당 코드처럼 기입해준다.
그다음 상단 render_template 옆에
, request, jsonify
를 넣어준다.
from flask import Flask, render_template, request, jsonify
app = Flask(__name__)
@app.route('/')
def home():
return render_template('index.html')
@app.route('/test', methods=['GET'])
def test_get():
title_receive = request.args.get('title_give')
print(title_receive)
return jsonify({'result': 'success', 'msg': '이 요청은 GET!'})
if __name__ == '__main__':
app.run('0.0.0.0', port=5000, debug=True)
그 다음 실행해보자

성공했으니 이 요청은 GET! 이라는 msg 를 가져오는지 콘솔창으로 확인해보자
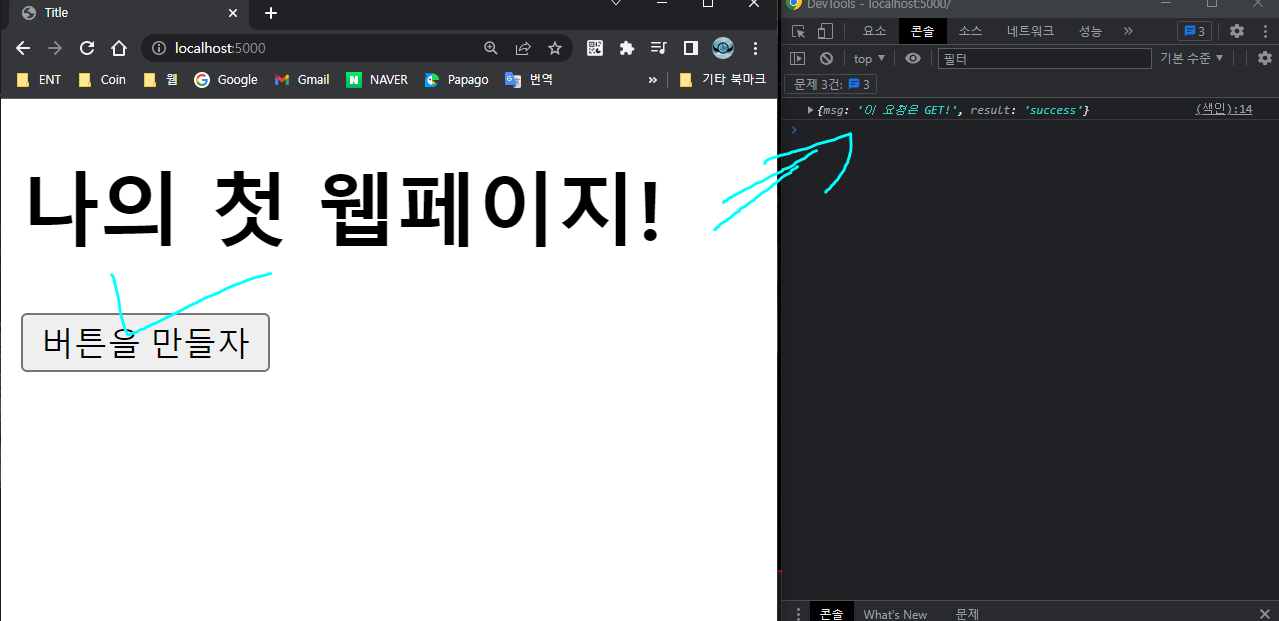
이것도 성공이다.
POST 요청 API 만들기
POST 요청 확인 AJAX 코드
▼
<script>
function hey() {
$.ajax({
type: "POST",
url: "/test",
data: {title_give: '봄날은간다'},
success: function (response) {
console.log(response)
}
})
}
</script>
해당 post 요청 확인 AJAX 코드를 기입하고
POST 요청 API 코드
▼
@app.route('/test', methods=['POST'])
def test_post():
title_receive = request.form['title_give']
print(title_receive)
return jsonify({'result':'success', 'msg': '이 요청은 POST!'})
POST 창구를 만들어주는 POST 요청 API 코드를 기입해준다.
실행!
이 요청은 POST 라는 msg 를 콘솔창에 보여준다.
여기서 마지막 하나만 더!
{msg: '이 요청은 POST!', result: 'success'}
이 콘솔창에 나온 구문을
msg 만 표현해보자
index.html HTML 파일에서
console.log(response['msg'])
로 바꿔주자
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script>
function hey() {
$.ajax({
type: "POST",
url: "/test",
data: {title_give: '봄날은간다'},
success: function (response) {
console.log(response['msg'])
}
})
}
</script>
</head>
<body>
<h1>나의 첫 웹페이지!</h1>
<button onclick="hey()">버튼을 만들자</button>
</body>
</html>
성공이다.
'📁𝐩𝐫𝐨𝐠𝐫𝐚𝐦𝐦𝐢𝐧𝐠𝐋𝐚𝐧𝐠𝐮𝐚𝐠𝐞 > Python' 카테고리의 다른 글
[Flask] 화성땅 공동구매 (2) 뼈대 준비하기 (1) | 2022.12.31 |
---|---|
[Flask] 화성땅 공동구매 (1) 프로젝트 세팅 (0) | 2022.12.31 |
[Flask] HTML 파일 주기 (0) | 2022.12.31 |
[Flask] 서버 만들기 (4) | 2022.12.30 |
[웹 스크래핑 크롤링] 웹스크래핑(크롤링) 연습 (0) | 2022.12.29 |